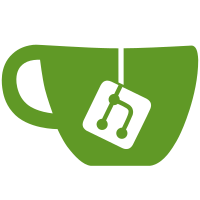
commit e48c78765ca1e2e5a68fd93bac7191eaf6918352 Author: Jordan Sherer <jordan@widefido.com> Date: Wed Jan 2 12:23:28 2019 -0500 Transition to persistent inbox for later retrieval Fixed issue with inbox items disappearing due to aging. commit 1df07595bf6507438c1488839f7a2075a432a1a1 Author: Jordan Sherer <jordan@widefido.com> Date: Wed Jan 2 09:23:28 2019 -0500 Filtered value and count queries for the inbox commit c93a93a1c43a65fae4a31ddeb40c77c53204bbdb Author: Jordan Sherer <jordan@widefido.com> Date: Tue Jan 1 22:58:07 2019 -0500 Initial cut of inbox storage
77 lines
2.0 KiB
C++
77 lines
2.0 KiB
C++
/**
|
|
* This file is part of JS8Call.
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
*
|
|
* (C) 2018 Jordan Sherer <kn4crd@gmail.com> - All Rights Reserved
|
|
*
|
|
**/
|
|
|
|
#include "Message.h"
|
|
|
|
Message::Message()
|
|
{
|
|
}
|
|
|
|
Message::Message(QString const &type, QString const &value):
|
|
type_{ type },
|
|
value_{ value }
|
|
{
|
|
}
|
|
|
|
Message::Message(QString const &type, QString const &value, QMap<QString, QVariant> const ¶ms):
|
|
type_{ type },
|
|
value_{ value },
|
|
params_{ params }
|
|
{
|
|
}
|
|
|
|
void Message::read(const QJsonObject &json){
|
|
if(json.contains("type") && json["type"].isString()){
|
|
type_ = json["type"].toString();
|
|
}
|
|
|
|
if(json.contains("value") && json["value"].isString()){
|
|
value_ = json["value"].toString();
|
|
}
|
|
|
|
if(json.contains("params") && json["params"].isObject()){
|
|
params_.clear();
|
|
|
|
QJsonObject params = json["params"].toObject();
|
|
foreach(auto key, params.keys()){
|
|
params_[key] = params[key].toVariant();
|
|
}
|
|
}
|
|
}
|
|
|
|
void Message::write(QJsonObject &json) const{
|
|
json["type"] = type_;
|
|
json["value"] = value_;
|
|
|
|
QJsonObject params;
|
|
foreach(auto key, params_.keys()){
|
|
params.insert(key, QJsonValue::fromVariant(params_[key]));
|
|
}
|
|
json["params"] = params;
|
|
}
|
|
|
|
QByteArray Message::toJson() const {
|
|
QJsonObject o;
|
|
write(o);
|
|
|
|
QJsonDocument d(o);
|
|
return d.toJson(QJsonDocument::Compact);
|
|
}
|