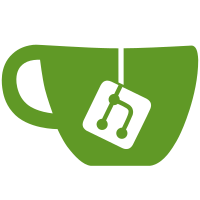
commit e48c78765ca1e2e5a68fd93bac7191eaf6918352 Author: Jordan Sherer <jordan@widefido.com> Date: Wed Jan 2 12:23:28 2019 -0500 Transition to persistent inbox for later retrieval Fixed issue with inbox items disappearing due to aging. commit 1df07595bf6507438c1488839f7a2075a432a1a1 Author: Jordan Sherer <jordan@widefido.com> Date: Wed Jan 2 09:23:28 2019 -0500 Filtered value and count queries for the inbox commit c93a93a1c43a65fae4a31ddeb40c77c53204bbdb Author: Jordan Sherer <jordan@widefido.com> Date: Tue Jan 1 22:58:07 2019 -0500 Initial cut of inbox storage
50 lines
922 B
C++
50 lines
922 B
C++
#ifndef INBOX_H
|
|
#define INBOX_H
|
|
|
|
/**
|
|
* (C) 2018 Jordan Sherer <kn4crd@gmail.com> - All Rights Reserved
|
|
**/
|
|
|
|
#include <QObject>
|
|
#include <QString>
|
|
#include <QPair>
|
|
#include <QVariant>
|
|
|
|
#include "vendor/sqlite3/sqlite3.h"
|
|
|
|
#include "Message.h"
|
|
|
|
|
|
class Inbox
|
|
{
|
|
public:
|
|
explicit Inbox(QString path);
|
|
~Inbox();
|
|
|
|
// Low-Level Interface
|
|
bool isOpen();
|
|
bool open();
|
|
void close();
|
|
QString error();
|
|
int count(QString type, QString query, QString match);
|
|
QList<QPair<int, Message>> values(QString type, QString query, QString match, int offset, int limit);
|
|
Message value(int key);
|
|
int append(Message value);
|
|
bool set(int key, Message value);
|
|
bool del(int key);
|
|
|
|
// High-Level Interface
|
|
int countUnreadFrom(QString from);
|
|
QPair<int, Message> firstUnreadFrom(QString from);
|
|
|
|
signals:
|
|
|
|
public slots:
|
|
|
|
private:
|
|
QString path_;
|
|
sqlite3 * db_;
|
|
};
|
|
|
|
#endif // INBOX_H
|